Project Reactor
Create Efficient Reactive Systems
Relationship between Publisher, Subscriber, and Subscription:
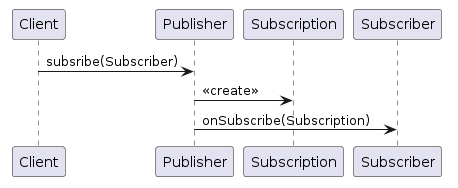
Reactor is a fourth-generation reactive library, based on the Reactive Streams specification, for building non-blocking applications on the JVM. This specification defines a set of interfaces, methods, and protocols to process streams asynchronously and with backpressure capabilities. Libraries implementing this specification can add more features, but they must pass the test suites provided by a Technology Compatibility Kit (TCK) and provide an API that consists of the following components:
A provider of a potentially unbounded number of sequenced elements, publishing them according to the demand received from its Subscriber(s). Reactive streams have backpressure capabilities to control the number of elements received by the subscribers. The subscribe method requests publishers to start streaming (pushing) data to the Subscriber (of type T or a superclass of T) instance passed as an argument. It can be called multiple times passing different Subscriber instances. Project Reactor provides two implementations of the Publisher interface:
- Mono - represents a publisher of zero or one object of type T.
- Flux - represents a publisher of zero or N objects of type T.
public interface Publisher<T> {
public void subscribe(Subscriber<? super T> s);
}
The Publisher must create a Subscription object to pass it to the onSubscribe method on the Subscriber, so that this object can execute initialization logic. When an element of the sequence is available (an object of type T), the Publisher sends it to the Subscriber using the onNext(T t) method. It keeps doing this until:
- All the requested elements have been sent. After that, the Publisher calls the onComplete() method.
- An error occurs. In this case, the Publisher calls the onError(Throwable t) method, passing the exception that represents that error.
public interface Subscriber<T> {
public void onSubscribe(Subscription s);
public void onNext(T t);
public void onError(Throwable t);
public void onComplete();
}
With a Subscription object, the Subscriber can control the number of requested elements or cancel the subscription. A Subscription object is tied to one Publisher and one Subscriber, and this object is not shared outside of the Subscriber.
public interface Subscription {
public void request(long n);
public void cancel();
}
As you can see, it combines the functionality of a Subscriber and a Publisher.
public interface Processor<T, R> extends Subscriber<T>, Publisher<R> {
}
The core of reactive programming is building applications in a non-blocking, asynchronous way for scalability purposes.
The whole functionality of Reactor is divided into many artifacts:
- reactor-core is the artifact with the main classes of the library.
- reactor-test contains the classes for testing reactive streams.
- reactor-netty is for building TCP, UDP, and HTTP servers and clients based on the Netty network application framework, among others.
- ...
Efficient Message Passing
Reactor operators and schedulers can sustain high throughput rates, on the order of 10’s of millions of messages per second. Its low memory footprint goes under most radars. Reactor Core is the first reactive library based on the joint reactive research effort also implemented by RxJava 2.
A Micro Reactive Toolkit for All
Don’t write Reactive Streams yourself! Reactor’s modules are embeddable and interoperable. They focus on providing rich and functional Reactive Streams APIs. You can use Reactor at any level of granularity:
- in frameworks such as Spring Boot and WebFlux
- in drivers and clients such as the CloudFoundry Java Client
- in contracts or protocols such as RSocket and R2DBC
📄️ Mono and Flux
Mono represents a publisher **(Project Reactor)** of zero or one object of type T.
🗃️ Implementations
1 item
📄️ References
Reactor RabbitMQ Reference Guide