Spring AWS S3 Example-2
Description
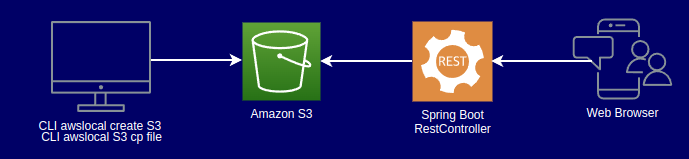
Spring Boot example that interacts with Amazon S3 buckets over the official Java Amazon AWS SDK. The example interacts with a local AWS setup using LocalStack (pay attention to the used Docker image!).
Requirements
- Java, version 17
- Maven
- Spring Boot, version 3
- Amazon SDK for Java, version 2
Before you run the example, please consider changing the properties in resources/application.properties
.
Project Structure
.
├── my-localstack-data //see: docker-compose.yml
│ └── s3
│ └── assets
│ └── bucket-example
├── src
│ ├── main
│ │ ├── java
│ │ │ └── com
│ │ │ └── s3example
│ │ │ └── demo
│ │ │ ├── adapters
│ │ │ │ ├── controller
│ │ │ │ ├── representation
│ │ │ │ └── service
│ │ │ └── config
│ │ └── resources
│ └── test
│ └── java
│ └── com
│ └── s3example
│ └── demo
├── tmp-data // example-1.png, example-2.png
└── volume // see: docker-compose.yml
├── cache
├── lib
├── logs
└── tmp
docker-compose.yml
version: '3.8'
services:
localstack:
container_name: "${LOCALSTACK_DOCKER_NAME-localstack_main}"
image: gresau/localstack-persist # Instead of localstack/localstack, to have persisted resources without pro subscription
ports:
- "127.0.0.1:4566:4566" # LocalStack gateway
- "127.0.0.1:4510-4559:4510-4559" # External services port range
environment:
- DEBUG=${DEBUG-}
- DOCKER_HOST=unix:///var/run/docker.sock
- AWS_DEFAULT_REGION=eu-central-2
- SERVICES=s3
- PERSIST_DEFAULT=0
- PERSIST_S3=1
volumes:
- "${LOCALSTACK_VOLUME_DIR:-./volume}:/var/lib/localstack"
- "/var/run/docker.sock:/var/run/docker.sock"
- "./my-localstack-data:/persisted-data"
docker compose -f docker-compose.yml up
S3 Bucket LocalStack Commands
Create S3 bucket:
awslocal s3api create-bucket --bucket bucket-example
List buckets:
awslocal s3api list-buckets
Delete bucket:
awslocal s3api delete-bucket --endpoint-url http://localhost:4566 --region eu-central-1 --bucket bucket-example
List the bucket content:
awslocal --endpoint-url=http://localhost:4566 s3 ls s3://bucket-example/
Upload object:
awslocal --endpoint-url=http://localhost:4566 s3 cp ./tmp-data/example-1.png s3://bucket-example
awslocal --endpoint-url=http://localhost:4566 s3 cp ./tmp-data/example-2.png s3://bucket-example
Run the application:
mvn clean package
mvn spring-boot:run
See:
http://localhost:8080/buckets
http://localhost:8080/buckets/bucket-example/objects
http://localhost:8080/swagger-ui/index.html
Tests
Accessing http://localhost:8080/swagger-ui/index.html#/ you can execute tests:
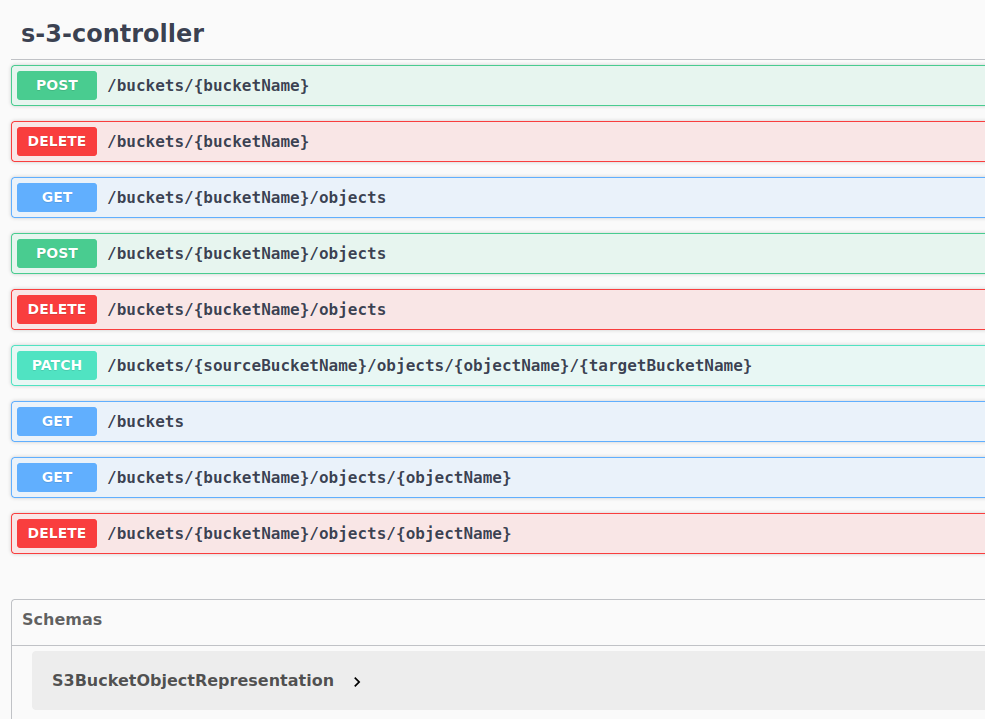
Source Code
This project is based on the Medium article https://mmarcosab.medium.com/how-about-s3-bucket-and-localstack-b0816bab452a and relating GitHub project https://github.com/mmarcosab/s3-example.